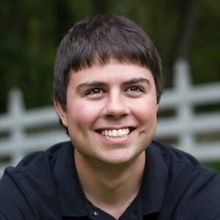
Dave Ceddia
Dave Ceddia - профессионально пишет программного обеспечение более 10 лет. React - его любимая технология. Автор книги "Pure React" и многих постов в блогах по теме разработки.
Вы не знаете Реакт. Но не из-за отсутствия попыток изучить его! Учебники, книги, курсы, подкасты, посты в блогах ... Вы все их читали или пытались это сделать. Вы следовали за всем этим, и, конечно, это имело хоть какой-то смысл, пока вы усердно воспроизводили каждый шаг, но только когда вы достигли конца, все, что вы якобы выучили, просто ... забылось или превратилось в огромное серое небытие: туман неопределенности...
React превратил вас из живого, дышащего человека, способного учиться и делать разные вещи, в пульсирующий знак вопроса:
И самый большой знак вопроса из всех ...
"Почему я не могу понять REACT ??"
Может быть, вы не старались изо всех сил ...?
Вернулись к одному из бесполезных уроков и постарались изо всех сил вбить его в свой мозг - снова?
Но эти уроки действительно не помогают.
Вместо того, чтобы делать вещи проще - вместо того, чтобы дать вам спокойную, ясную, простую структуру - они накапливают больше путаницы.
Они не учат вас React.
Они мешают все это с Webpack, Babel, Redux, React Router, Apollo, Next.js, Gatsby, SSR, Firebase, Docker… Плюс, конечно, ES6, с его проимисами и его странным синтаксическим сахаром, таким как => и {destructuring: {things }}…
Эти курсы обещают, что все это будет стоить того, потому что вы научитесь «создавать полное приложение React от начала до конца!»
Но проблема этого подхода в том, что вы очень мало узнаете о многих вещах. Когда вы собираетесь применить свои знания в реальном мире, вы попадаете в огромные дыры в ваших знаниях и понимании.
Я запускаю новый, улучшенный и продвинутый курс Pure React
Я взял лучшие части книги Pure React и превратил их в серию кратких, самостоятельных видеоуроков, которые помогут вам учиться глубже, дальше и быстрее.
Каждый урок проходил боевые испытания с живой аудиторией - всего более 500 человек!
Каждый видеоурок был профессионально отредактирован, проверен и протестирован, чтобы обеспечить учебные материалы высочайшего качества, которые вы сможете использовать для развития своих навыков веб-разработчика.
Уроки короткие, легко усваиваемые и точные. И все они построены вокруг небольших, достижимых проектов. Мы начинаем с малого и продвигаемся вверх.
Вы создадите серию автономных проектов:
Вот что вы получите:
Pure React включает в себя:
Современный JavaScript для React
Не можете сказать, какие части кода являются React, а какие JavaScript? Прежде чем вы сможете эффективно работать с современными фреймворками, такими как React, вы должны понять язык, на котором он основан (или, по крайней мере, дела пойдут гораздо более гладко!). В этом модуле вы изучите основной синтаксис современного JavaScript, необходимый для работы с React.
Мы расскажем о стрелочных функциях и о том, как они отличаются от обычных функций, а также о операторах ... rest и ... spread. Затем мы перейдем к immutability и узнаем почему это важно (очень важно понимать, чтобы избежать странных ошибок в ваших приложениях). Вы узнаете, как использовать функции map, filter и reduce и в заключение мы узнаем, как импортировать и экспортировать модули.
React: Введение
Добро пожаловать в Реакт! В этом модуле вы напишите свое первое приложение React и узнаете, как создавать приложения с использованием синтаксиса React JSX. Мы намеренно начинаем с простого, с жестко закодированными данными и без «состояния», о котором нужно беспокоиться. После прохождения этих уроков вы поймете достаточно основополагающих концепций React, чтобы иметь возможность создавать статические приложения, как если бы вы работали с простым HTML.
Классический React c Class Components
До этого момента все компоненты, которые мы создали, были без сохранения состояния и в значительной степени статичны. В этом модуле вы узнаете, как писать компоненты класса в React для обработки состояния, что позволит нам создавать интерактивные компоненты.
Вплоть до React 16.8 классы были единственным способом добавления состояния к компонентам. Сегодня у нас есть хуки, которые позволяют нам добавлять состояние в компоненты функции без написания класса. Так что вы можете задаться вопросом, зачем вообще учить классы?
Реальность такова, что существует множество кода React, существовавшего до Hooks, и многие компании не любят переписывать код, который уже работает. Полное понимание компонентов класса даст вам преимущество при поиске задания React, а также поможет при поиске ответов в StackOverflow и тому подобном.
Использование React Hooks
В React версии 16.8 добавлены хуки, набор функций, которые позволяют добавлять состояние и побочные эффекты к компонентам функций. В этом модуле вы узнаете, как использовать хуки в ваших компонентах! Начиная с useState, мы расскажем, как добавить простое и сложное состояние в ваши функции. Оттуда мы рассмотрим использование useReducer для обработки более сложных состояний. Вы также научитесь писать многоразовые пользовательские хуки. И мы поговорим о том, как хуки работают за кулисами, чтобы демистифицировать «магию»
API Запросы в React
Вскоре после начала работы с React вы захотите получить некоторые данные из внешнего источника, будь то ваш собственный API или чужой. В этом модуле мы создадим зоомагазин, в котором мы сможем просмотреть список домашних животных в центре принятия, добавить новых домашних животных, отредактировать их имена и фотографии и удалить.
Мы начнем со статического приложения с жестко закодированными данными и реорганизуем его по частям, пока все данные не поступают и не отправляются с сервера API.
React Context для состояния приложения
Во многих случаях команды используют Redux как фактический выбор для управления состоянием. Но иногда Redux бывает излишним. В этих случаях React Context API может полностью удовлетворить ваши потребности.
В этом модуле мы создадим простой почтовый клиент, использующий React Context API для передачи данных по всему приложению. Вы узнаете, как создать контекст, как глубоко передавать данные через приложение, не пропуская повсюду пропсы, и как группировать связанные данные и логику, используя простые компоненты-оболочки. Мы также рассмотрим практические приложения, такие как использование Context для отображения уведомлений и управления ими, а также способы повышения производительности с помощью Context.
[Начало 2020] Async React c Suspense
Suspense и Concurrent в корне меняют способы разработки приложений React. Pure React будет обновлен, чтобы отразить эти изменения!
Этот модуль будет доступен после запуска в начале 2020 года.
[Начало 2020] Создайте полное приложение React
Давайте объединим все, что мы узнали, в одно мощное реальное приложение React!
Этот модуль будет доступен после завершения Suspense и Concurrent в начале 2020 года.
Интервью с разработчиками
Покупатели Pure React Pro получат доступ к видео-интервью с экспертами отрасли по темам, которые помогут вам выйти за пределы Pure React.
Архив сримов
Я часами преподавал весь этот материал живой аудитории и оттачивал его, прежде чем записывать урок. Эти сессии были записаны, но записи не были доступны для общественности до сих пор. В качестве специального бонуса, включенного в пакет Pro, вы получите доступ к просмотру этих живых событий!
https://github.com/dceddia/pure-react-lessons-code
Dave Ceddia - профессионально пишет программного обеспечение более 10 лет. React - его любимая технология. Автор книги "Pure React" и многих постов в блогах по теме разработки.
Обычное состояние React просто. Вы вызываете setState, компонент повторно рендерится, и… вот и все. Это относится к одному компоненту. Никаких скачков между файлами. Легко. Redux совсем другой зверь.
Топовый фреймворк - простым языком. Время начать изучение React!Минимальный порог входных знаний!
Все что ты хочешь знать про React. Основная часть курса включает 108 уроков общей продолжительностью 16 часов. И это без учета бонусов, в которых содержится ВСЯ необходимая теоретическая база для овладения React.js с полного нуля (включая основы JavaScript)!
Узнайте, как защитить свои приложения React для реального мира. Я провел годы, работая в базах кода React, где безопасность является главной заботой. Я также провел почти три года, работая в Auth0, где я многое узнал об аутентификации и безопасности. Я хотел бы научить вас всему, что я знаю, о том, как защитить ваши приложения React, чтобы вам не приходилось тратить все это время на то, чтобы разобраться в этом.